Overview
Form Designers with knowledge
of JavaScript can add business rules and customized actions to their
Field2Base Forms. You can access the Forms Designer script editor by clicking on the Script icon in the Home tab. The JavaScript Sample Form 7.0 attached at the bottom of this article contains examples of all available script calls.
Events
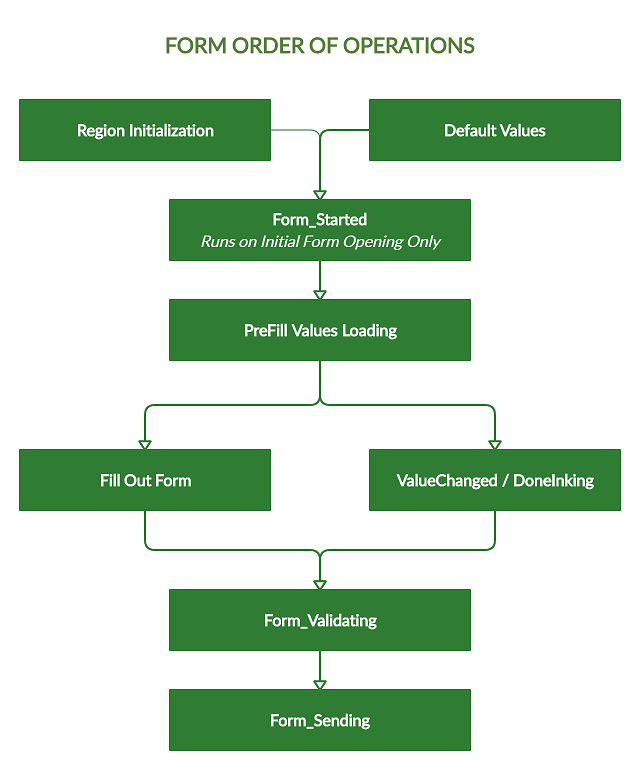
Event raised when the user starts work on a new form.
- function Form_Started() {
- var objUser = Field2Base.getUser();
- var userFullName = objUser.firstName + " " + objUser.lastName;
- Form.getRegion("Page3@Form_Started_Message").setValue("The Form Draft has been opened by " + userFullName + ".");
- }
Event raised before the form gets sent. You can use objValidationLog.addRegionErrorMessage to display a validation error and prevent the user from sending the form until conditions are met.
objValidationLog.addRegionErrorMessage(region, strMessage)
The Validation Log records problems discovered during form validation. If the log contains any entries then the form cannot be sent. This method will only work in the Form_Validating() function.
- function Form_Validating(objValidationLog) {
- var rgnValidation = Form.getRegion("Page3@Form_Validating_Required");
- if (!rgnValidation.getValue()) {
- objValidationLog.addRegionErrorMessage(rgnValidation, "Please fill out the Form Validating Region before sending.");
- }
- }
Event raised after the user specifies that the form is ready to be sent. Be cautious about script in the Sending event. You generally should not make changes to form data because the user may not have a chance to see and validate the new values. If the user opens and resends a form (for example, as part of a workflow), the Sending event will be raised again. Users may also have an option to resend a form without opening it, in which case the Sending event may not be raised before re-transmitting form data. The Sending event may also be raised multiple times before sending the form if the client gives the user a way to cancel the transmission after the event has already been raised.
- function Form_Sending() {
- Form.getRegion("Page3@Form_Sending_Message").setValue("The Form has been sent.");
- }
Event raised when the value is changed in any Region on the form.
- function Form_DataChanged(objArgs) {
- var rgnCurrent = objArgs.getRegion();
- var regionName = rgnCurrent.getName();
- if (regionName.indexOf("Rating") >= 0) {
- var regionValue = rgnCurrent.getValue();
- var lineNum = regionName.slice(7);
- var pageNum = rgnCurrent.getOwningPage().getUserPageNumber();
- var rgnWarning = Form.getRegion("Page" + pageNum + "@Warning_" + lineNum);
- if (regionValue <= 2) {
- rgnWarning.setValue("! LOW");
- } else {
- rgnWarning.clearValue();
- }
- }
- }

Note: When adding script to the Form_DataChanged event, you may encounter data processing errors if you have a large number of regions with initial values. In order to avoid this error and unnecessary data processing, you should create IF statements to narrow down the targeted regions before manipulating region values. For example, in the script above, the Region Name must contain "Rating" before any values are changed inside the body of the IF statement, as opposed to being globally declared within the Form_DataChanged event.
PageNum_Region_ValueChanged(objArgs)
Event raised when a single Region's value is changed.
- function Page4_Text_To_Change_ValueChanged(objArgs) {
- var count = Form.getRegion("Page4@Count_hidden").getValue();
- var pluralTime = "time";
- count++;
- if (count > 1) {
- pluralTime = "times";
- }
- Form.getRegion("Page4@Count_hidden").setValue(count);
- Form.getRegion("Page4@Value_Changed_Count").setValue("The region has been changed " + count + " " + pluralTime + ".");
- }
PageNum_Region_DoneInking(objArgs)
Event raised 3 seconds after a user stops inking in a Region.
- function Page4_Signature_DoneInking(objArgs) {
- if (objArgs.getRegion().getHasStrokes()) {
- var timestamp = new Date().getTime();
- var convertedTimestamp = new Date(timestamp);
- var month = (convertedTimestamp.getMonth() + 1);
- var day = convertedTimestamp.getDate();
- var year = convertedTimestamp.getFullYear();
- Form.getRegion("Page4@Signature_Date").setValue("Signed on: " + month + "/" + day + "/" + year);
- } else {
- Form.getRegion("Page4@Signature_Date").clearValue();
- }
- }
Note: Global variables do not work consistently across all platforms. We do not recommend using global variables. In cases where global variables are needed, you can use a hidden region to store a value that can be used like a global variable in your script. To hide a region, right click on the region and choose "Remove from Page".
The Form class is the root node in the form object tree. There is a global instance called Form that is the object for the user's current eForm.
Returns the name of the Form Template.
- function getFormName() {
- var formName = Form.getName();
- Form.getRegion("Page5@FormName").setValue(formName);
- }
Form.getPagesCount()
Returns the number of pages in the Form.
- function getFormPageCount() {
- var pageCount = Form.getPagesCount();
- Form.getRegion("Page5@PageCount").setValue(pageCount);
- }
Form.getPageByPageName(strPageName)
Returns the page with the given page name.
- function changeRegionValueUsingPageName() {
- var pg1 = Form.getPageByPageName("Page Uno");
- pg1.getRegion("GetPageByName").setValue("This Region has been changed using Form.getPageByPageName().");
- }
Form.getPageByUserPageNumber(pgNum)
Returns the page with the given user page number.
- function changeRegionValueUsingPageNumber() {
- var pg5 = Form.getPageByUserPageNumber(5);
- pg5.getRegion("GetPageByNumber").setValue("This Region has been changed using Form.getPageByUserPageNumber().");
- }
Form.getPageAtIndex(pgNum)
Returns the page at the given position at the given index. The index is the 0-based position of the page in the Form.
- function getIndex() {
- var pgIndex4 = Form.getPageAtIndex(4);
- var pageIndex = Form.getPageIndex(pgIndex4);
- pgIndex4.getRegion("PageIndex").setValue(pageIndex);
- }
Form.getPageIndex(page)
Returns an integer of the index of the given page. The index is the 0-based position of the page in the Form.

Note: The index, unlike the user page number, will change if a page is inserted before it.
- function getIndex() {
- var pgIndex4 = Form.getPageAtIndex(4);
- var pageIndex = Form.getPageIndex(pgIndex4);
- pgIndex4.getRegion("PageIndex").setValue(pageIndex);
- }
Returns a string with the Payment Account for the Form.

Note: If there is not a Payment Account explicitly set using the Form.setPaymentAccount() call previously in the Form, a blank or undefined value will be returned.
- function getPaymentAccount() {
- var paymentAccount = Form.getPaymentAccount();
- Form.getRegion("Page1@CurrentPaymentAccount").setValue(paymentAccount);
- }
Form.insertPage(page, pgIndex)
Inserts a given page into the Form at the given index.
- function insertOrderPage() {
- var pgOrderOfOps = Form.getPageByUserPageNumber(2);
- var pgNewOrderOfOps = pgOrderOfOps.duplicateTemplate();
- Form.insertPage(pgNewOrderOfOps, Form.getPagesCount());
- }
Returns the given Region.
- function changeRegionValueUsingForm() {
- Form.getRegion("Page5@FormGetRegion").setValue("This Region has been changed using Form.getRegion().");
- }
Locks all Regions in the Form.
- function lockAll() {
- Form.lockRegionValues();
- }
Sets the Payment Account for the Form using a string representing the Payment Account Name setup in the Portal.
- function setPaymentAccount() {
- var paymentAccount = Form.getRegion("Page1@NewPaymentAccount").getValue();
- Form.setPaymentAccount(paymentAccount);
- Form.getRegion("Page1@CurrentPaymentAccount").setValue(Form.getPaymentAccount());
- }
Unlocks all Regions in the Form.
- function unlockAll() {
- Form.unlockRegionValues();
- }
Page
The Page class is a container for Regions.
Page.getName()
Returns the name of a page.
- function getPageName() {
- var pageName = Form.getPageByUserPageNumber(6).getName();
- Form.getRegion("Page6@PageName").setValue(pageName);
- }
Page.setName(newName)
Sets the name of a page.
- function changePageName() {
- var newPageName = Form.getRegion("Page6@NewPageName").getValue();
- Form.getPageByUserPageNumber(6).setName(newPageName);
- }
Page.getUserPageNumber()
Returns the page number of a page.
- function getPageNumber() {
- var pageNumber = Form.getPageAtIndex(5).getUserPageNumber();
- Form.getRegion("Page" + pageNumber +"@PageNumber").setValue(pageNumber);
- }
Page.setVisible(boolean)
Sets whether a page is visible or not.
- function toggleVisibility() {
- var pgOrderOfOps = Form.getPageByUserPageNumber(2);
- var isVisible = pgOrderOfOps.getVisible();
- if (isVisible) {
- pgOrderOfOps.setVisible(false);
- } else {
- pgOrderOfOps.setVisible(true);
- }
- }
Page.getVisible()
Returns boolean for whether or not a page is visible or not.
- function viewIsVisible() {
- var pgOrderOfOps = Form.getPageByUserPageNumber(2);
- var isVisible = pgOrderOfOps.getVisible();
- Form.getRegion("Page7@Visibility").setValue(isVisible);
- }
Page.duplicateTemplate()
Returns a copy of the page template (background and Regions). The page data is not copied.
- function insertOrderPage() {
- var pgOrderOfOps = Form.getPageByUserPageNumber(2);
- var pgNewOrderOfOps = pgOrderOfOps.duplicateTemplate();
- Form.insertPage(pgNewOrderOfOps, Form.getPagesCount());
- }
Page.getRegion(rgnName)
Returns the specified Region on a page.
- function changeRegionValueUsingPage() {
- var pg7 = Form.getPageByUserPageNumber(7);
- pg7.getRegion("PageGetRegion").setValue("This Region has been changed using Page.getRegion().");
- }
Page.lockRegionValues()
Locks all Regions on a page.
- function lockAllPage() {
- var pg7 = Form.getPageByUserPageNumber(7);
- pg7.lockRegionValues();
- }
Page.unlockRegionValues()
Unlocks all Regions on a page.
- function unlockAllPage() {
- var pg7 = Form.getPageByUserPageNumber(7);
- pg7.unlockRegionValues();
- }
Region
The Region class represents fields on the Form.
Region.setValue(newValue)
Sets the value of the Region.
- function changeRegionValue() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- rgnExample.setValue("This Region has been set using Region.setValue().");
- }
Region.getValue()
Returns the value of the Region.
- function getRegionValue() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- var exampleRegionValue = rgnExample.getValue();
- View.showDialog("The value of the top region is: " + exampleRegionValue, 0);
- }
Region.clearValue()
Clears the value of the Region.
- function clearRegionValue() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- rgnExample.clearValue();
- }
Region.lockValue()
Locks the Region.
- function lockRegion() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- rgnExample.lockValue();
- }
Region.unlockValue()
Unlocks the Region.
- function unlockRegion() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- rgnExample.unlockValue();
- }
Region.getIsLocked()
Returns a boolean of whether the Region is locked or unlocked.
- function checkRegionLocked() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- var regionLocked = rgnExample.getIsLocked();
- Form.getRegion("Page8@RegionCheckLocked").setValue(regionLocked);
- }
Region.setIsRequired(boolean)
Sets whether the Region is required or not.
- function requireRegion() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- rgnExample.setIsRequired(true);
- }
- function doNotRequireRegion() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- rgnExample.setIsRequired(false);
- }
Region.isRequired()
Returns a boolean of whether the Region is required or not.
- function checkRegionRequired() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- var checkRequired = rgnExample.isRequired();
- Form.getRegion("Page8@RegionCheckRequired").setValue(checkRequired);
- }
Region.getName()
Returns the name of the Region.
- function getRegionName() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- var regionName = rgnExample.getName();
- Form.getRegion("Page8@RegionGetName").setValue(regionName);
- }
Region.getOwningPage()
Returns the page that the Region is on.
- function getRegionPage() {
- var rgnExample = Form.getRegion("Page8@RegionExample");
- var page = rgnExample.getOwningPage();
- var pageName = page.getName();
- Form.getRegion("Page8@RegionGetPageName").setValue(pageName);
- }
CAMERA REGIONS
Region.getHasImage()
Returns a boolean of whether the Camera Region has an image or not.
- function checkCameraImage() {
- var rgnCameraExample = Form.getRegion("Page9@CameraExample");
- var checkImage = rgnCameraExample.getHasImage();
- Form.getRegion("Page9@CameraCheckImage").setValue(checkImage);
- }
Region.clearImage()
Clears the current image from the Camera Region.
- function clearCameraImage() {
- var rgnCameraExample = Form.getRegion("Page9@CameraExample");
- rgnCameraExample.clearImage();
- }
CHECKBOX REGIONS
Region.setChecked(boolean)
Sets the Checkbox Region to checked or unchecked.
- function toggleCheckboxRegion() {
- var rgnCheckboxExample = Form.getRegion("Page9@CheckboxExample");
- var checked = rgnCheckboxExample.getChecked();
- if (!checked) {
- rgnCheckboxExample.setChecked(true);
- } else {
- rgnCheckboxExample.setChecked(false);
- }
- }
Region.getChecked()
Returns a boolean of whether the Checkbox Region is currently checked or unchecked.
- function checkCheckboxRegion() {
- var rgnCheckboxExample = Form.getRegion("Page9@CheckboxExample");
- var checked = rgnCheckboxExample.getChecked();
- Form.getRegion("Page9@CheckboxCheck").setValue(checked);
- }
Region.hasDatasourceList()
Returns boolean of whether the Datasource Region has a list or not.
- function checkDatasourceRegion() {
- var rgnDatasourceExample = Form.getRegion("Page10@DatasourceExample");
- var hasList = rgnDatasourceExample.hasDatasourceList();
- Form.getRegion("Page10@DatasourceCheckList").setValue(hasList);
- }
Region.getDatasourceList()
Returns an array of the list for the Datasource Region.
- function getList() {
- var rgnDatasourceExample = Form.getRegion("Page10@DatasourceExample");
- var list = rgnDatasourceExample.getDatasourceList();
- Form.getRegion("Page10@DatasourceList").setValue(list);
- }
Region.setDatasourceList(array)
Sets the list for the Datasource Region. The parameter must be an array of string values.
- function replaceList() {
- var rgnDatasourceExample = Form.getRegion("Page10@DatasourceExample");
- var newListArr = ["new item 1","new item 2", "new item 3"];
- rgnDatasourceExample.setDatasourceList(newListArr);
- }
GPS REGIONS
Region.takeLatestCoordinate()
Returns the most recent location as the value for a GPS region.

Note: May be a stale value if the Form has stopped receiving location updates or it may not have any value if the Form has not yet received any location updates.
- function getGPSCoordinates() {
- var rgnGPS = Form.getRegion("Page10@GPSCoordinates");
- rgnGPS.takeLatestCoordinate();
- }
Region.getCoordinates()
Returns an object containing the latitude, longitude, elevation, and timestamp for the most recent location. Only latitude and longitude are guaranteed to be present; elevation and timestamp may be null. When timestamp is present, it is a UTC value formatted as an ISO 8601 string.

Note: This method only works in eForm Templates Version 7.0 and higher.
- function getGPSObject() {
- var rgnGPSObject = Form.getRegion("Page10@GPSObject");
- var latitude = rgnGPSObject.getCoordinates().latitude;
- var longitude = rgnGPSObject.getCoordinates().longitude;
- var elevation = rgnGPSObject.getCoordinates().elevation;
- var timestamp = rgnGPSObject.getCoordinates().timestamp;
- Form.getRegion("Page10@GPSDisplay").setValue("Latitude: " + latitude + "\nLongitude: " + longitude + "\nElevation: " + elevation + "\nTimestamp: " + timestamp);
- }
PEN REGIONS
Region.getHasStrokes()
Returns a boolean of whether the Pen Region contains any ink strokes or not.
- function checkPenRegion() {
- var rgnPenExample = Form.getRegion("Page11@PenExample");
- var hasStrokes = rgnPenExample.getHasStrokes();
- Form.getRegion("Page11@PenCheck").setValue(hasStrokes);
- }
Region.clearStrokes()
Clears all ink strokes from the Pen Region.
- function clearPenRegion() {
- var rgnPenExample = Form.getRegion("Page11@PenExample");
- rgnPenExample.clearStrokes();
- }
The View class exposes control features for an eForm editing view. There is a single global instance called View that lets script access the client's currently active view if there is one. If a client supports more than one open eForm view then this instance will manipulate whichever view the client has specified as the active view, which may change as the user works with the form.
View.showDialog(strMessage, intDialogType, callback)
Shows a popup message box with a message and a chosen button configuration. The button configuration specifies which buttons are available for the user to select.
Possible button configurations are:
0 OK
1 OK, Cancel
3 Yes, No, Cancel
4 Yes, No
The return value indicates which button the user chose. Values are:
1 OK
2 Cancel
6 Yes
7 No
- function view0() {
- View.showDialog("Are You Sure?", 0, "dialogResultHandler0");
- }
- function dialogResultHandler0(result){
- var returnValue;
- if (result == 1) {
- returnValue = "OK";
- }
- Form.getRegion("Page12@Dialog0").setValue("You clicked: " + returnValue);
- }
- function view1() {
- View.showDialog("Are You Sure?", 1, "dialogResultHandler1");
- }
- function dialogResultHandler1(result){
- var returnValue;
- if (result == 1) {
- returnValue = "OK";
- } else if (result == 2) {
- returnValue = "Cancel";
- }
- Form.getRegion("Page12@Dialog1").setValue("You clicked: " + returnValue);
- }
- function view3() {
- View.showDialog("Are You Sure?", 3, "dialogResultHandler3");
- }
- function dialogResultHandler3(result){
- var returnValue;
- if (result == 2) {
- returnValue = "Cancel";
- } else if (result == 6) {
- returnValue = "Yes";
- } else if (result == 7) {
- returnValue = "No";
- }
- Form.getRegion("Page12@Dialog3").setValue("You clicked: " + returnValue);
- }
- function view4() {
- View.showDialog("Are You Sure?", 4, "dialogResultHandler4");
- }
- function dialogResultHandler4(result){
- var returnValue;
- if (result == 6) {
- returnValue = "Yes";
- } else if (result == 7) {
- returnValue = "No";
- }
- Form.getRegion("Page12@Dialog4").setValue("You clicked: " + returnValue);
- }
View.openURL(strURL)
Opens a URL in a web browser. For web sites, the URL string must include the "http://" or "https://" for the method to run correctly. The default browser will be used to open the URL specified.
Note: This method only works in eForm Templates Version 7.0 and higher.
Set boolean to true to open the URL in a Web View within the Mobile Forms app. False opens the URL in an external browser (default).
View.showUserPageNumber(pgNum)
Navigates to the specified page.
- function goToMainMenu() {
- View.showUserPageNumber(1);
- }
Field2Base
The Field2Base class provides information about the current F2B user's session. There is a single global instance called Field2Base.
Sends the current draft. This initiates validation and other send processing. Calling this method is equivalent to the user clicking Send in the App UI. All validation and on-send processing occurs as it normally would.
- function submitForm() {
- Field2Base.send();
- }
Field2Base.closeDraft(boolPrompt)
Closes the current draft and uses the boolean parameter to show (true) or not show (false) the Save Draft prompt. If using 'false', the draft is automatically discarded.
This function cannot be called from the Form_Sending Event, and it does not factor in the Save Draft Prompt config; it uses the boolean parameter instead, overriding the Save Draft Prompt config.
- function closeDraftPrompt() {
- Field2Base.closeDraft(true);
- }
- function closeDraftNoPrompt() {
- Field2Base.closeDraft(false);
- }
Field2Base.getUser()

Note: This method only works in eForm Templates Version 7.0 and higher.
Returns an object with User information.
- function getUserInfo() {
- var objUser = Field2Base.getUser();
- var firstName = objUser.firstName;
- var lastName = objUser.lastName;
- var email = objUser.email;
- var username = objUser.username;
- // Personnel must be enabled for the company for the following details to be available
- var ID = objUser.personnelID;
- var title = objUser.jobTitle;
- var type = objUser.personnelType;
- var defaultLocation = objUser.defaultLocation;
- var defaultApproval = objUser.defaultGroup;
-
- Form.getRegion("Page13@UserInfo").setValue("First name: " + firstName + " Last name: " + lastName + "\nEmail: " + email + "\nUsername: " + username + "\nID: " + ID + " Title: " + title + " Type: " + type + "\nLocation: " + defaultLocation + " Approval: " + defaultApproval);
- }
Field2Base.getUsername()
Returns the username of the logged-in User.
- function getUsername() {
- Form.getRegion("Page13@Username").setValue(Field2Base.getUsername());
- }
Field2Base.getUserFirstName()
Returns the first name of the logged-in User.
- function getFirstName() {
- Form.getRegion("Page13@FirstName").setValue(Field2Base.getUserFirstName());
- }
Field2Base.getUserLastName()
Returns the last name of the logged-in User.
- function getLastName() {
- Form.getRegion("Page13@LastName").setValue(Field2Base.getUserLastName());
- }
Field2Base.getUserPhoneNumber()
Returns the phone number of the logged-in User.
There is no validation on the Phone Number field in the User Profile so other information (employee ID, etc...) can be used to be pulled into forms.
- function getPhoneNumber() {
- Form.getRegion("Page14@PhoneNumber").setValue(Field2Base.getUserPhoneNumber());
- }
Field2Base.getUserEmail()
Returns the e-mail address of the logged-in User.
- function getEmail() {
- Form.getRegion("Page14@Email").setValue(Field2Base.getUserEmail());
- }
Field2Base.getLocationNames()
Note: This method only works in eForm Templates Version 7.0 and higher.
Note: Locations must be enabled for the company.
Returns an array of the Location names.
- function getLocations() {
- var locations = Field2Base.getLocationNames();
- Form.getRegion("Page14@Locations").setDatasourceList(locations);
- }
Field2Base.getLocationDetails(LocationName)
Returns an object with the details for the specified Location.
- function getDetails() {
- var locations = Field2Base.getLocationNames();
- var location1Obj = Field2Base.getLocationDetails(locations[0]);
- var name = location1Obj.name;
- var type = location1Obj.type;
- var contact = location1Obj.contactName;
- var contactEmail = location1Obj.contactEmail;
- var contactPhone = location1Obj.contactPhone;
- var group = location1Obj.groupName;
- var requirements = location1Obj.requirements;
- Form.getRegion("Page14@LocationDetails").setValue("Name: " + name + " Type: " + type + "\nContact: " + contact + " Email: " + contactEmail + " Phone: " + contactPhone + "\nGroup: " + group + "\nRequirements: " + requirements);
- }
Field2Base.getProjectId()
Returns the unique id of the Form's Folder.
- function getFolderID() {
- Form.getRegion("Page14@FolderID").setValue(Field2Base.getProjectId());
- }
Field2Base.getProjectName()
Returns the name of the form's Folder.
- function getFolderName() {
- Form.getRegion("Page14@FolderName").setValue(Field2Base.getProjectName());
- }
Environment and Locale
The Environment class provides information about the current environment where the app is running and returns the current Locale object of the Environment which can only be used in conjunction with the Locale properties (Code, Language, and Region).

Note: This is not implemented on Web App.
Environment.getCurrentLocale().getCode()
Returns the full language and territory code of the Locale instance. Typically, the two letter language code and the two letter territory code that will be separated by a hyphen or an underscore. For example, a device running the US English Language would have a code of "en_US" or "en-US". The codes returned are dependent on the Operating System so it is NOT recommended as the best way to determine a User's Language due to the lack of uniform returned values.
- function getPlatformCode() {
- var code = Environment.getCurrentLocale().getCode();
- Form.getRegion("Page15@LocaleCode").setValue(code);
- }
Environment.getCurrentLocale().getLanguage()
Returns the language code of the Locale instance. Typically, the two letter language code will be returned for this property. For that reason, this is the recommended way to determine a User's Language.
- function getPlatformLanguage() {
- var lang = Environment.getCurrentLocale().getLanguage();
- Form.getRegion("Page15@LocaleLanguage").setValue(lang);
- }
Environment.getCurrentLocale().getRegion()
Returns the region (or territory) code of the Locale instance. Typically, the two letter territory code will be returned for this property so values like "US" for the United States or "GB" for Great Britain will be returned.
- function getPlatformRegion() {
- var region = Environment.getCurrentLocale().getRegion();
- Form.getRegion("Page15@LocaleRegion").setValue(region);
- }